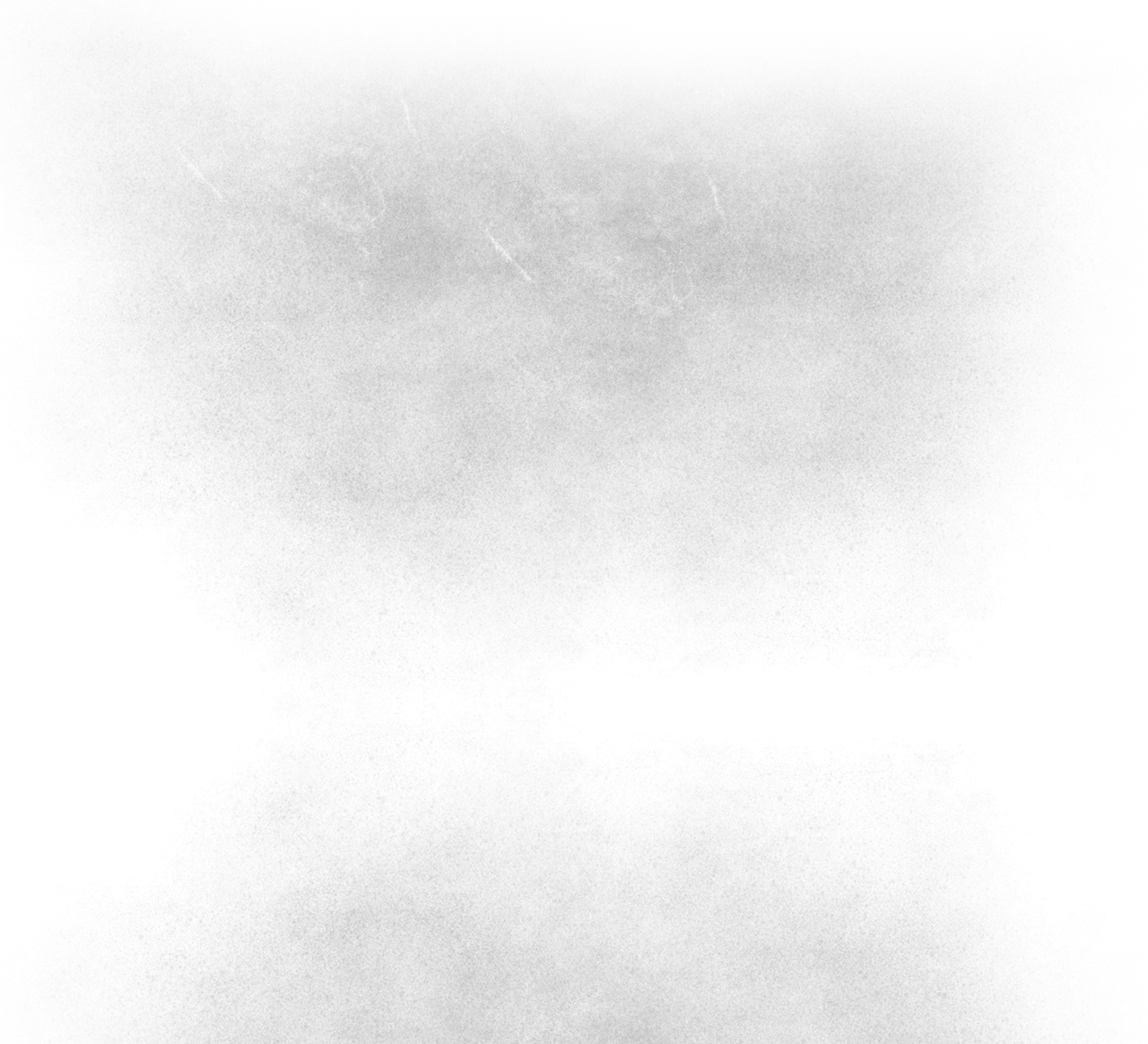
​​Chap 18/100: Terrain with 3 SLOP Textures and with a Detail Texture​
​
What I will Learn here?:
​On this tutorial ​​we will learn how to render the terrain with a detail texture based on the z-distance
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​Note:
Try to move the camera down and check the detail
​
​Let's check our current main source tree, except the LIBs:
​
Added source on White:
​​
​│ Applicationclass.cpp
│ Applicationclass.h
│ counter.h
│ main.cpp
│ main.h
│
├───camera
│ cameraClass.cpp
│ cameraClass.h
│ frustumClass.cpp
│ frustumClass.h​
│ lightClass.cpp
│ lightClass.h
│ positionClass.cpp
│ positionClass.h
│ RenderFrustumClass.cpp
│ RenderFrustumClass.h
│
├───game
│ playerClass.cpp
│ playerClass.h​
│​
├───graphics
│ renderTextureClass.cpp
│ renderTextureClass.h
│ spriteClass.cpp
│ spriteClass.h​
│ textClass.cpp
│ textClass.h
│ textFontClass.cpp
│ textFontClass.h
│
├───input
│ inputClass.cpp
│ inputClass.h
│
├───loader
│ objModelV2Class.cpp
│ objModelV2Class.h
│
├───shader
│ shaderClass.cpp
│ shaderClass.h
│
├───system
│ dx11Class.cpp
│ dx11class.h
│ SystemClass.cpp
│ SystemClass.h
│ xml_loader.cpp
│ xml_loader.h​
│​
​└───terrain
bitmapclass.cpp
bitmapclass.h
Minimapclass.cpp
Minimapclass.h
quadtreeClass.cpp
quadtreeClass.h
terrainClass.cpp
terrainClass.h
terrainManagerClass.cpp
terrainManagerClass.h
​
​​​(main.h) ​
- Set for Chapter 18
​
018terrain_fog_slope_detail.hlsl shader file using Fog an the Slop and a detail texture:
​
​
////////////////////////////////////////////////////////////////////////////////
// Filename: 018terrain_fog_slope_detail.hlsl
////////////////////////////////////////////////////////////////////////////////
#include "000cbuffer.hlsl"
//////////////
// TYPEDEFS //
//////////////
// VERTEX:
struct VSIn
{
float4 position : POSITION;
float4 tex : TEXCOORD0; //CH04 - TEXTURE
float3 normal : NORMAL; //CH12 - LIGHT
float4 color : COLOR; //CH13 - MIX COLOR (Not used on this Example)
};
// PIXEL:
struct PSIn
{
float4 position : SV_POSITION;
float4 tex : TEXCOORD0;
float3 normal : NORMAL; //CH12 - LIGHT
float4 color : COLOR; //CH13 - MIX COLOR
float fogFactor : FOG; //CH16 - FOG
float4 depthPosition : TEXCOORD1; //CH18
float4 inputPosition : TEXCOORD2; //CH18
};
/////////////
// GLOBALS //
/////////////
//CH17&CH18 - There are three textures we will be using for the three different degrees of slope that we want to handle.
Texture2D grassTexture : register(t0); //CH17
Texture2D slopeTexture : register(t1); //CH17
Texture2D rockTexture : register(t2); //CH17
Texture2D detailTexture : register(t3); //CH18
SamplerState SampleType; //CH05 - TEXTURE
////////////////////////////////////////////////////////////////////////////////
// Vertex Shader
////////////////////////////////////////////////////////////////////////////////
PSIn MyVertexShader018terrain_fog_slope_detail(VSIn input)
{
PSIn output;
float4 cameraPosition; //FOG
input.position.w = 1.0f; // Change the position vector to be 4 units for proper matrix calculations.
output.inputPosition = input.position;
output.position = mul(input.position, WVP); // Calculate the position of the vertex against the world, view, and projection matrices.
output.tex = input.tex; // Store the texture coordinates for the pixel shader.
//LIGHT:
output.normal = normalize(mul(input.normal, (float3x3)worldMatrix));// Calculate the normal vector against the world matrix only.
output.color = input.color; // Send the color map color into the pixel shader.
//FOG:
cameraPosition = mul(input.position, WV); // FOG: Calculate the camera position.
output.fogFactor = saturate((fogEnd - cameraPosition.z) / (fogEnd - fogStart)); //CH16 - FOG - Calculate linear fog:
//CH18 - Store the position value in a second input value for depth value calculations.
output.depthPosition = cameraPosition;
return output;
}
////////////////////////////////////////////////////////////////////////////////
// Pixel Shader
////////////////////////////////////////////////////////////////////////////////
float4 MyPixelShader018terrain_fog_slope_detail(PSIn input) : SV_TARGET
{
float4 textureColor; //CH05 - TEXTURE
float lightIntensity; //CH12 - LIGHT
float4 color = ambientColor;
float4 grassColor; //CH17 - SLOP
float4 slopeColor; //CH17
float4 rockColor; //CH17
float slope; //CH17
float blendAmount; //CH17
float4 detailColor; //CH18
float detailBrightness; //CH18
float4 fogColor = float4(0.5f, 0.5f, 0.5f, 1.0f); //FOG
if (input.fogFactor > 0) {
//CH17 - Start by sampling all three textures.
//------------------------------------------------------------------------------------------------------
// Sample the grass color from the texture using the sampler at this texture coordinate location.
grassColor = grassTexture.Sample(SampleType, input.tex.xy) * 0.75f;
// Check if the depth value is close to the screen, if so we will apply the detail texture.
if( input.depthPosition.z < 6) // TRY TO: COMMENT ME!
{
// Sample the pixel color from the detail map texture using the sampler at this texture coordinate location.
detailColor = detailTexture.Sample(SampleType, input.tex.zw);
// Set the brightness of the detail texture.
detailBrightness = 2;
// Combine the ground texture and the detail texture. Also multiply in the detail brightness.
grassColor = grassColor * detailColor * detailBrightness;
}
// For using with extra Color also:
grassColor = 2*saturate (grassColor * input.color); // TRY TO: COMMENT ME!
// Sample the slope color from the texture using the sampler at this texture coordinate location.
slopeColor = slopeTexture.Sample(SampleType, input.tex.xy);
// Sample the rock color from the texture using the sampler at this texture coordinate location.
rockColor = rockTexture.Sample(SampleType, input.tex.xy);
//CH17 - Now determine the slope for this pixel, which is just one subtracted from the Y normal.
//------------------------------------------------------------------------------------------------------
// Calculate the slope of this point.
slope = 1.0f - input.normal.y;
//CH17
// Since we have the slope we can now use it in some if statements and determine which texture to use based on the slope of the pixel.
// To make things look smooth we do a linear interpolation between the textures so the transition between each one
// isn't a sharp line in the terrain.
// Determine which texture to use based on height.
if(slope < 0.2f)
{
blendAmount = slope / 0.2f;
textureColor = lerp(grassColor, slopeColor, blendAmount);
}
if((slope < 0.7f) && (slope >= 0.2f))
{
blendAmount = (slope - 0.2f) * (1.0f / (0.7f - 0.2f));
textureColor = lerp(slopeColor, rockColor, blendAmount);
}
if(slope >= 0.7f)
{
textureColor = rockColor;
}
//LIGHT:
lightIntensity = saturate(dot(input.normal, lightDirection));// Calculate the amount of light on this pixel.
color += (diffuseColor * lightIntensity); // Determine the final diffuse color based on the diffuse color and the amount of light intensity.
color = color * textureColor; // The texture pixel is combined with the light color to create the final color result.
// 2- The fog color equation does a linear interpolation between the texture color and the fog color based on the fog factor input value.
color = input.fogFactor * color + (1.0 - input.fogFactor) * fogColor; // FOG: Calculate the final color using the fog effect equation.
​
return color;
} else {
clip (-1.0); return (float4)0; // this pixel is too far away (on fog), SKIP it! CLIP IF: (x is less than zero)
}
}
​
​​
​​​​Project Code:
​​​​​​​http://woma.no-ip.org/woma/WoMA_PartII_Chap18.zip
​​
​What's next?
On next tutorial we will see how to Render a the terrain with Multi Textures Mapping


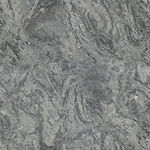
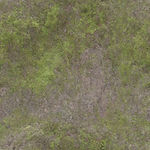
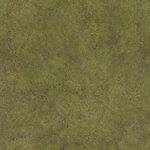




