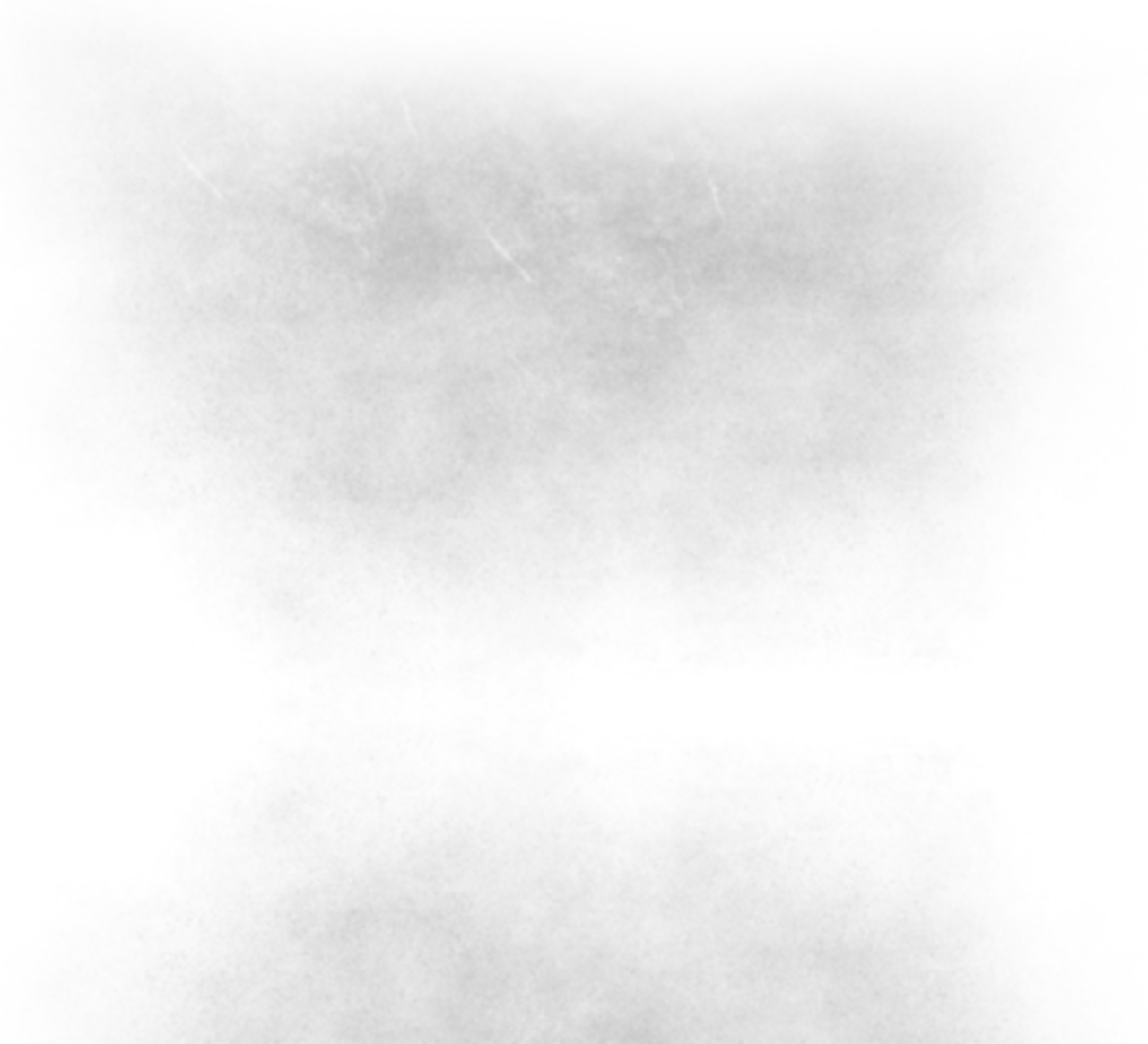
​​Chap 21/100: Terrain with Small UV Bump Mapping​​​
​
What I will Learn here?:
​On this tutorial ​​we will learn how to render the terrain with a detail texture based on the z-distance
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​Note:
Try to move the camera down and check the detail of the bump mapping effect
​
​Let's check our current main source tree, except the LIBs:
​
Added source on White:
​​
​│ Applicationclass.cpp
│ Applicationclass.h
│ counter.h
│ main.cpp
│ main.h
│
├───camera
│ cameraClass.cpp
│ cameraClass.h
│ frustumClass.cpp
│ frustumClass.h​
│ lightClass.cpp
│ lightClass.h
│ positionClass.cpp
│ positionClass.h
│ RenderFrustumClass.cpp
│ RenderFrustumClass.h
│
├───game
│ playerClass.cpp
│ playerClass.h​
│​
├───graphics
│ renderTextureClass.cpp
│ renderTextureClass.h
│ spriteClass.cpp
│ spriteClass.h​
│ textClass.cpp
│ textClass.h
│ textFontClass.cpp
│ textFontClass.h
│
├───input
│ inputClass.cpp
│ inputClass.h
│
├───loader
│ objModelV2Class.cpp
│ objModelV2Class.h
│
├───shader
│ shaderClass.cpp
│ shaderClass.h
│
├───system
│ dx11Class.cpp
│ dx11class.h
│ SystemClass.cpp
│ SystemClass.h
│ xml_loader.cpp
│ xml_loader.h​
│​
​└───terrain
bitmapclass.cpp
bitmapclass.h
Minimapclass.cpp
Minimapclass.h
quadtreeClass.cpp
quadtreeClass.h
terrainClass.cpp
terrainClass.h
terrainManagerClass.cpp
terrainManagerClass.h
​
​​​(main.h) ​
- Set for Chapter 21
​
021terrain.hlsl shader file using Fog an the Slop and a detail texture:
​
​
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
// Filename: 021terrain.hlsl
////////////////////////////////////////////////////////////////////////////////
#include "000cbuffer.hlsl"
//////////////
// TYPEDEFS //
//////////////
struct VSIn
{
float4 position : POSITION;
float4 tex : TEXCOORD0; //CH04 - TEXTURE
float3 normal : NORMAL; //CH12 - LIGHT
float4 color : COLOR; //CH13 - MIX COLOR
float4 texMapping : TEXCOORD1; //CH19 - Big Mapping
float3 tangent : TANGENT; //CH21
float3 binormal : BINORMAL; //CH21
};
struct PSIn
{
float4 position : SV_POSITION;
float4 tex : TEXCOORD0;
float3 normal : NORMAL;
float3 tangent : TANGENT;
float3 binormal : BINORMAL;
float4 depthPosition : TEXCOORD1;
};
//////////////
// TEXTURES //
//////////////
//Texture2D colorTexture : register(t0);
//Texture2D normalTexture : register(t1);
//CH17&CH18 - There are three textures we will be using for the three different degrees of slope that we want to handle.
Texture2D grassTexture : register(t0); //CH17
Texture2D slopeTexture : register(t1); //CH17
Texture2D rockTexture : register(t2); //CH17
Texture2D detailTexture : register(t3); //CH18
Texture2D textureMappingTexture : register(t4); //CH19
Texture2D colorTexture/*sidewalkTexture*/ : register(t5); //CH19
Texture2D sandTexture : register(t6); //CH19
Texture2D mudTexture : register(t7); //CH19
Texture2D alphaMappingTexture: register(t8); //CH20
Texture2D normalTexture/*sidewalkBumpTexture*/: register(t9); //CH21
//////////////
// SAMPLERS //
//////////////
SamplerState SampleType;
////////////////////////////////////////////////////////////////////////////////
// Vertex Shader
////////////////////////////////////////////////////////////////////////////////
PSIn MyVertexShader021terrain(VSIn input)
{
PSIn output;
// Change the position vector to be 4 units for proper matrix calculations.
input.position.w = 1.0f;
output.position = mul(input.position, WVP); // Calculate the position of the vertex against the world, view, and projection matrices.
output.tex = input.tex; // Store the texture coordinates for the pixel shader.
//LIGHT:
output.normal = normalize(mul(input.normal, (float3x3)worldMatrix));// Calculate the normal vector against the world matrix only.
// Store the position value in a second input value for depth value calculations.
output.depthPosition = mul(input.position, WV); //FOG: Calculate the camera position.
output.tangent = normalize(mul(input.tangent, (float3x3)worldMatrix)); // Calculate the tangent vector against the world matrix only and then normalize the final value.
output.binormal = normalize(mul(input.binormal, (float3x3)worldMatrix));// Calculate the binormal vector against the world matrix only and then normalize the final value.
return output;
}
////////////////////////////////////////////////////////////////////////////////
inline float3 normapMapFunc(float4 bumpMap, PSIn input)
////////////////////////////////////////////////////////////////////////////////
{
float3 bumpNormal; //CH21
if(input.depthPosition.z < 10) // Bump only on closer textures (Faster version for the rest)
{
bumpMap = (bumpMap * 2.0f) - 1.0f; //Expand the range of the normal value from (0, +1) to (-1, +1).
bumpNormal = input.normal + bumpMap.x * input.tangent +
bumpMap.y * input.binormal; //Calculate the normal from the data in the bump map.
bumpNormal = normalize(bumpNormal); //Normalize the resulting bump normal.
} else {
bumpNormal = input.normal;
}
return bumpNormal;
}
////////////////////////////////////////////////////////////////////////////////
inline float3 lightFunc(float3 bumpNormal, float4 textureColor)
////////////////////////////////////////////////////////////////////////////////
{ float4 color = ambientColor;
float lightIntensity = saturate(dot(bumpNormal, lightDirection)); // Calculate the amount of light on this pixel.
color += (diffuseColor * lightIntensity);
return color * textureColor; // The texture pixel is combined with the light color to create the final color result.
}
////////////////////////////////////////////////////////////////////////////////
// Pixel Shader
////////////////////////////////////////////////////////////////////////////////
float4 MyPixelShader021terrain(PSIn input) : SV_TARGET
{
float4 textureColor; //CH05 - TEXTURE
float4 bumpMap; //CH21 Aux
float3 bumpNormal; //CH21 Aux
textureColor = colorTexture.Sample (SampleType, input.tex.zw); // Sample the texture pixel at this location.
bumpMap = normalTexture.Sample(SampleType, input.tex.zw); // Sample the pixel in the bump map.
/*
bumpMap = (bumpMap * 2.0f) - 1.0f; // Expand the range of the normal value from (0, +1) to (-1, +1).
bumpNormal = input.normal +
bumpMap.x * input.tangent + bumpMap.y * input.binormal; // Calculate the normal from the data in the bump map.
bumpNormal = normalize(bumpNormal); // Normalize the resulting bump normal.
*/
bumpNormal = normapMapFunc(bumpMap, input);
/*
//LIGHT WITH BUMP:
lightIntensity = saturate(dot(bumpNormal, lightDirection));// Calculate the amount of light on this pixel.
color += (diffuseColor * lightIntensity);
color = color * textureColor; // The texture pixel is combined with the light color to create the final color result.
*/
return float4 (lightFunc(bumpNormal, textureColor), 1);
}​
​
​​
​​​​Project Code:
http://woma.no-ip.org/woma/WoMA_PartII_Chap21.zip
​​
​What's next?
On next tutorial we will see how to Render a the terrain with Multi and Alpha Textures Mapping and with Bump Map.
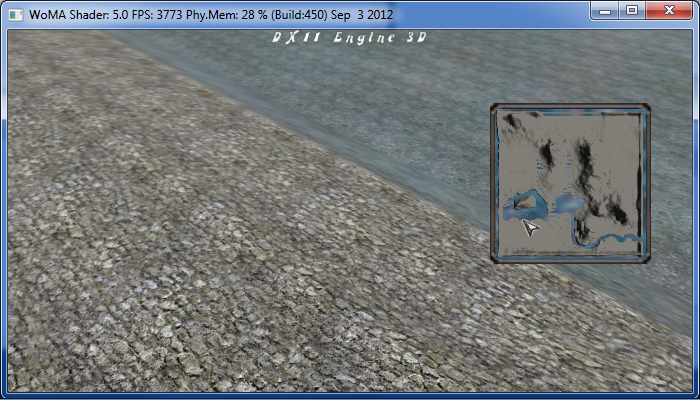
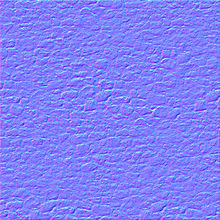
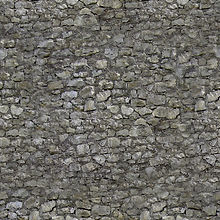




